C++でLCDに文字を表示
LCD1602はカナも表示できるので、その実験をしよう
/******************************************************************************************** *Filename : i2c_lcd.cpp *Description : test iic 1602 lcd *Author : Alan *Website : www.osoyoo.com *Update : 2017/07/03->2019/5/21 cpp update ********************************************************************************************/ #include <iostream> #include <wiringPi.h> #include <wiringPiI2C.h> #include <cstring> using namespace std; int LCDAddr = 0x27;//IIc LCD address int BLEN = 1;//1--open backlight.0--close backlight int fd;//linux file descriptor //writ a word(16 bits) to LCD void write_word(int data){ int temp = data; if ( BLEN == 1 ) temp |= 0x08; else temp &= 0xF7; wiringPiI2CWrite(fd, temp); } //send command to lcd void send_command(int comm){ int buf; // Send bit7-4 firstly buf = comm & 0xF0; buf |= 0x04; // RS = 0, RW = 0, EN = 1 write_word(buf); delay(2); buf &= 0xFB; // Make EN = 0 write_word(buf); // Send bit3-0 secondly buf = (comm & 0x0F) << 4; buf |= 0x04; // RS = 0, RW = 0, EN = 1 write_word(buf); delay(2); buf &= 0xFB; // Make EN = 0 write_word(buf); } //send data to lcd void send_data(int data){ int buf; // Send bit7-4 firstly buf = data & 0xF0; buf |= 0x05; // RS = 1, RW = 0, EN = 1 write_word(buf); delay(2); buf &= 0xFB; // Make EN = 0 write_word(buf); // Send bit3-0 secondly buf = (data & 0x0F) << 4; buf |= 0x05; // RS = 1, RW = 0, EN = 1 write_word(buf); delay(2); buf &= 0xFB; // Make EN = 0 write_word(buf); } //initialize the lcd void init(){ send_command(0x33); // Must initialize to 8-line mode at first delay(5); send_command(0x32); // Then initialize to 4-line mode delay(5); send_command(0x28); // 2 Lines & 5*7 dots delay(5); send_command(0x0C); // Enable display without cursor delay(5); send_command(0x01); // Clear Screen wiringPiI2CWrite(fd, 0x08); } //clear screen void clear(){ send_command(0x01); //clear Screen } //Print the message on the lcd void write(int x, int y, char* data){ int addr, i; int tmp; if (x < 0) x = 0; if (x > 15) x = 15; if (y < 0) y = 0; if (y > 1) y = 1; // Move cursor addr = 0x80 + 0x40 * y + x; send_command(addr); //tmp = strlen(data); for (; *data != '\0'; data++){ send_data(*data); } } //Print the message on the lcd void writekana(int x, int y, char* data){ int addr, i; int tmp; if (x < 0) x = 0; if (x > 15) x = 15; if (y < 0) y = 0; if (y > 1) y = 1; // Move cursor addr = 0x80 + 0x40 * y + x; send_command(addr); //tmp = strlen(data); for (; *data != '\0'; data++){ *data |= 0x80; send_data(*data); } } void print_info() { cout << "\n"; cout << "|***************************|\n"; cout << "| I2C 1602 LCD test |\n"; cout << "| --------------------------|\n"; cout << "| | LCD | | Pi |\n"; cout << "| --------------------------|\n"; cout << "| | GND | connect to | GND |\n"; cout << "| | VCC | connect to | 5V |\n"; cout << "| | SDA | connect to | SDA.1|\n"; cout << "| | SCL | connect to | SCL.1|\n"; cout << "| --------------------------|\n"; cout << "| OSOYOO|\n"; cout << "|***************************|\n"; cout << "Program is running...\n"; cout << "Press Ctrl+C to end the program\n"; } int main(){ //init I2C char sdata[100]; fd = wiringPiI2CSetup(LCDAddr); init(); print_info(); strcpy(sdata,"6@6E"); writekana(0, 0, sdata); strcpy(sdata,"VRY6E"); writekana(0, 1, sdata); delay(3000); clear(); while(1){ strcpy(sdata,"This is Lesson13"); write(0,0,sdata); strcpy(sdata,"IIC LCD Test"); write(0,1,sdata); delay(1000); } return 0; }
ASCIIで指定した文字に0x80をORしてむりやりカナに変えてみました
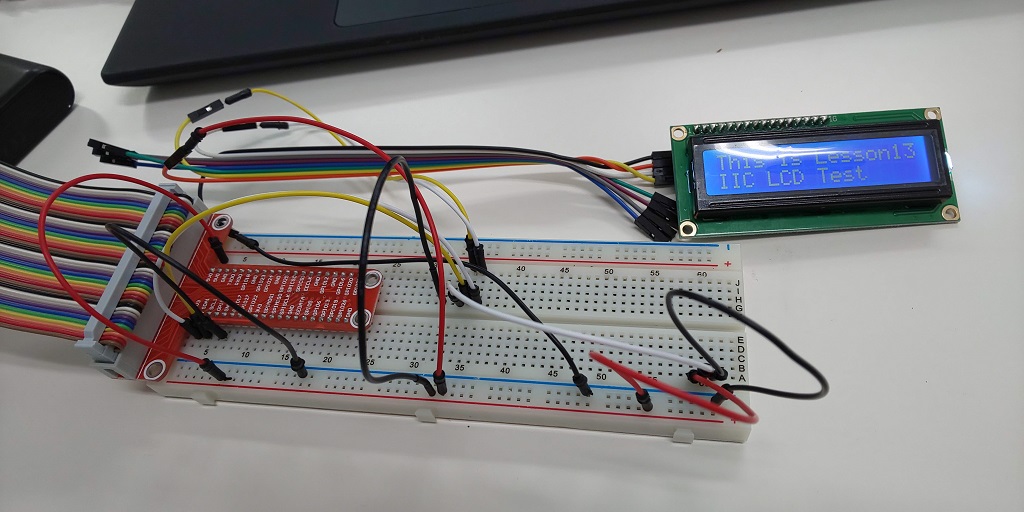