C++でA/Dコンバータの値を取り込む
MCP3008でボリュームの抵抗の変化を読み込む
/* * Success version * gcc mcp3002.c -omcp3002 -lwiringPi -DMCP3002 * Read MCP3002/MCP3204 using wiringPiSPIDataRW */ #include <iostream> #include <iomanip> #include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <wiringPi.h> #include <wiringPiSPI.h> using namespace std; #define DEBUG 0 #define SPI_CHANNEL 0 // /dev/spidev0.0 //#define SPI_CHANNEL 1 // /dev/spidev0.1 int main(int argc, char **argv){ int retCode; int i; int a2dChannel = 0; // analog channel int a2dVal = 0; float a2dVol = 0; float Vref = 3.3; #ifdef MCP3002 unsigned char data[2]; #endif #ifdef MCP3204 unsigned char data[3]; #endif if (argc == 2) { cout << "argv[1]=",argv[1],"\n"; a2dChannel=atoi(argv[1]); } // SPI channel 0 を 1MHz で開始。 =>200k if (wiringPiSPISetup(SPI_CHANNEL, 200000) < 0) { cout << "SPISetup failed:\n"; } for(i=0;i<20;i++) { #ifdef MCP3002 data[0] = 0b01100000 |( ((a2dChannel & 0x03) << 4)); // first byte transmitted -> start bit data[1] = 0; // third byte transmitted....don't care if(DEBUG) cout << "[MCP3002]data[write]=" << hex << data[0] << "-" << hex << data[1] <<"\n"; retCode=wiringPiSPIDataRW (SPI_CHANNEL,data,sizeof(data)); if(DEBUG) cout << "[MCP3002]wiringPiSPIDataRW=" << retCode << "\n"; if(DEBUG) cout << "[MCP3002]data[read]=" << hex << data[0] << "-" << hex << data[1] << "\n"; a2dVal = (data[0]<< 8) & 0b1100000000; //first 2 bit a2dVal |= (data[1] & 0xff); a2dVol = (float)a2dVal/1023 * Vref; #endif #ifdef MCP3204 data[0] = 0b00000110 |( ((a2dChannel & 0x04) >> 2)); // first byte transmitted -> start bit -> (SGL/DIF = 1, D2=0) data[1] = 0b00000000 |( ((a2dChannel & 0x03) << 6)); // second byte transmitted -> (D1=D0=0) data[2] = 0; // third byte transmitted....don't care if(DEBUG) cout << "[MCP3204]data[write]=" << hex << data[0] << "-" << hex << data[1] << "\n"; retCode=wiringPiSPIDataRW (SPI_CHANNEL,data,sizeof(data)); if(DEBUG) cout << "[MCP3004]wiringPiSPIDataRW=" << retCode << "\n"; if(DEBUG) cout << "[MCP3204]data[read]=" << hex << data[0] << "-" << hex << data[1] << "-" << hex << data[2]; a2dVal = (data[1]<< 8) & 0b111100000000; //first 4 bit a2dVal |= (data[2] & 0xff); a2dVol = (float)a2dVal/4095 * Vref; #endif cout << "a2dVal=" << a2dVal << "\n"; cout << "a2dVol=" << a2dVol << "[V]\n"; sleep(1); } }
ソースはMCP3002のものですが、MCP3008でも使えます。ただし結果は3002方が正しく出ます
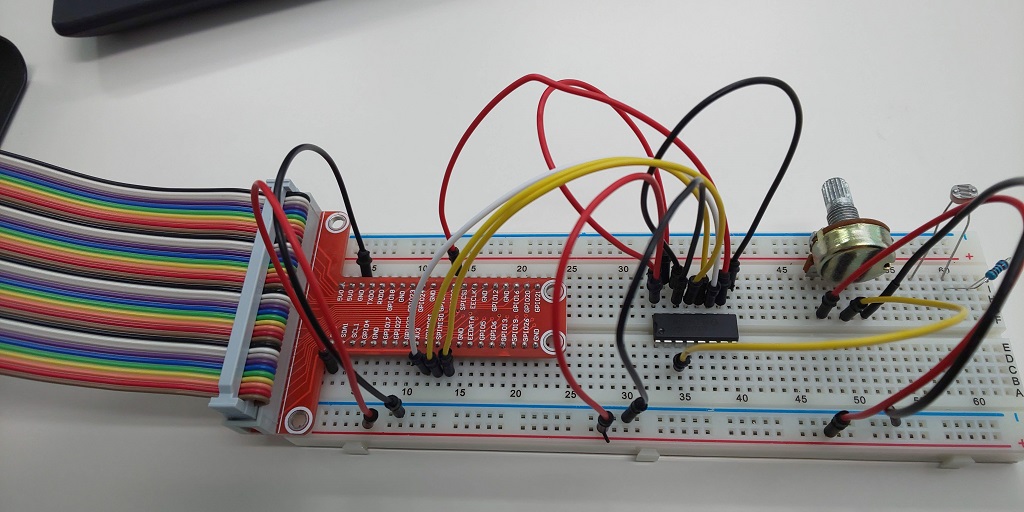